Getting Started with API Calls
Getting Started is Fast and Easy
- Join as a Developer - get your authentication keys
-
Signing a Bringg API Request - apply your Authentication keys to an API call
Join as a Developer
For Bringg API Calls, joining as a developer gets you the authentication keys you need:
- the access_token
- the secret_key
Before you start, you will have to register as a Developer:
- Verify your email address via registration email we sent to you. To resend the verification email use Company Settings and click on Resend Confirmation Email button.
- Go to Company Settings and click on Become a developer button.
- Fill out the Become a developer form.
- Go to Developer and copy your access_token and secret_key (you will have one pair for production and one for test).
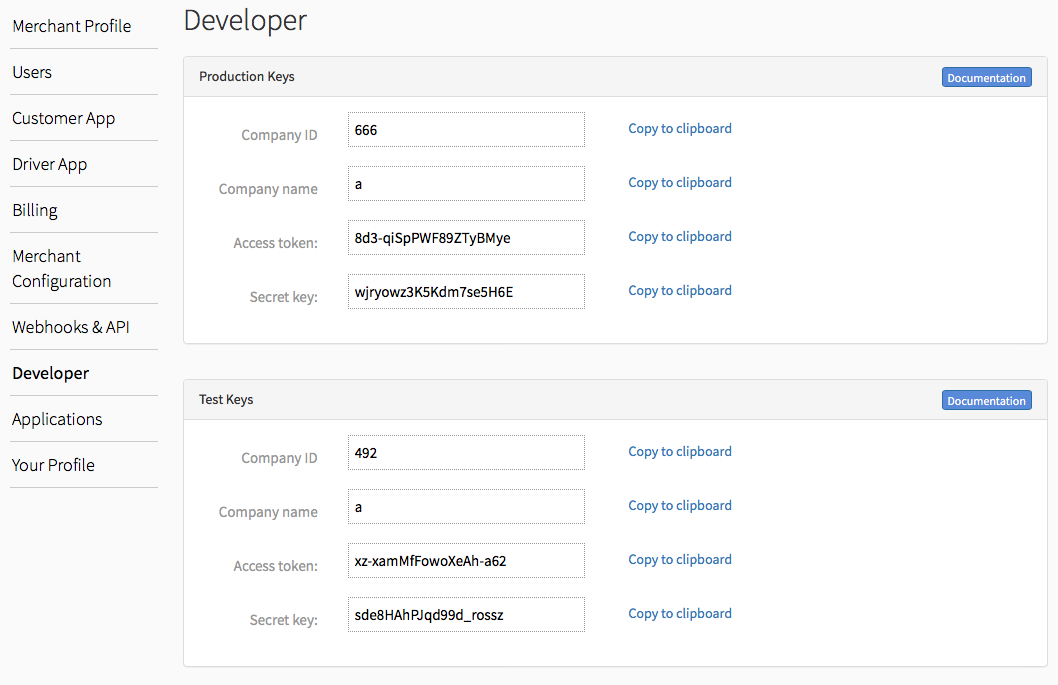
To Sign Bringg API Call
Signing a Bringg API Call requires:
- your parameters
- your Bring access-token (public key)
- your Bring secret-key (private key)
- the current time (in UNIX format)
To sign a Bringg API request:
- Add the current time (in UNIX format) and your access-token to your parameter list
- URL encode the parameter list
- Encrypt the parameter list using EmacSHA1 and your secret-key
You can also use our Tester to verify your signature
This example is taken from our Ruby client implementation. You can also look at the Javascript or PHP examples. for additional help or ask us to help with your own technology.
Example
You are signing a Bringg API request to Create Order (Task) that includes:
- customer_id = "174"
- title = "this is just a test"
Your authentication uses:
- access-token = "12345"
- secret-key = "67890"
1. Build Your Parameter List
Add each of the parameters to the parameter list:
customer_id
title
time stamp (in UNIX format)
access-token
params = {"customer_id" => 174, "title" => "this is just a test"}
params[:timestamp] ||= Time.now.to_i
params[:access_token] ||= "12345"
var params = JSON.parse('{"customer_id": 174, "title": "this is just a test"}');
params.timestamp = Date.now();
params.access_token = "12345";
<?php
$data = array(
'customer_id' => 174,
'title' => "this is just a test"
);
$data["access_token"] = "12345";
$data["timestamp"] = date('Y-m-d H:i:s');
?>
2. Create Your Request Signature
URL encode the parameter list to a query string
- Encrypt the query string using EmacSHA1 and the secret-key
query_params = params.to_query
params.merge(signature: OpenSSL::HMAC.hexdigest("sha1", "67890", query_params))
var query_params = '';
for (var key in params) {
var value = params[key];
if (query_params.length > 0) {
query_params += '&';
}
query_params += key + '=' + encodeURIComponent(value);
}
params.signature = CryptoJS.HmacSHA1(query_params, "67890").toString();
<?php
$secret_key = "67890";
$query_string = http_build_query($data);
$signature = hash_hmac("sha1", $query_string, $secret_key);
$data["signature"] = $signature;
?>
3. The Signature Is Your Query String
The signature includes your entire parameter list encrypted. Use the signature as your query string in the API URL.
Make a Bringg API Call
Example - Creating Your First Customer
You are executing a Bringg API request to the Create Customer endpoint which will create your first Customer with the following details:
- Name: Rose M. Scudder
- Address: 715 Oliverio Drive, Moundridge, KS 67107
- Phone: 620-680-9382
- E-mail: [email protected]
Your authentication uses:
- access-token = "12345"
- secret-key = "67890"
Getting Your access_token and secret-key
Join as a Developer to get your access_token and secret_key.
1. Add Your access_token & Timestamp To Your Parameters
var params = JSON.parse('{"name": "Rose M. Scudder", "address": "715 Oliverio Drive, Moundridge, KS 67107", "phone": "620-680-9382", "email": "[email protected]"}');
params.timestamp = Date.now();
params.access_token = "12345";
params = {"name" => "Rose M. Scudder", "address" => "715 Oliverio Drive, Moundridge, KS 67107", "phone" => "620-680-9382", "email" => "[email protected]"}
params[:timestamp] ||= Time.now.to_i
params[:access_token] ||= "12345"
2. URL Encode Your Parameters To A Query String
URL Encoding
For more information about URL Encoding, see URL encoded.
var query_params = '';
for (var key in params) {
var value = params[key];
if (query_params.length > 0) {
query_params += '&';
}
query_params += key + '=' + encodeURIComponent(value);
}
query_params = params.to_query
3. Sign the Query String
HmacSHA1 Encryption
For more information about HmacSHA1 encryption, see HmacSHA1.
params.signature = CryptoJS.HmacSHA1(query_params, "67890").toString();
params.merge(signature: OpenSSL::HMAC.hexdigest("sha1", "67890", query_params))
Authentication is Done
Make sure you have now 3 new parameters: timestamp, access_token and signature. You may want to verify your signature by using our Signature Tester.
4. Make the Bringg API Call
Create HTTP POST request to an endpoint:
request.open('POST', 'https://developer-api.bringg.com/partner_api/customers', true);
request.setRequestHeader('Content-type', 'application/json');
request.send(JSON.stringify(params));
uri = URI("http://api.bringg.com/partner_api/customers")
req = Net::HTTP::Post.new(uri, initheader = {'Content-Type' =>'application/json'})
req.body = params.to_json
res = Net::HTTP.start(uri.hostname, uri.port) do |http|
http.request(req)
end
Here is an example of the data that would have been returned:
{
"success": true,
"customer": {
"id": 1,
"name": "Rose M. Scudder",
"address": "715 Oliverio Drive, Moundridge, KS 67107",
"phone": "620-680-9382",
"email": "[email protected]"
}
}
Congratulations!
You have just made your first Bringg API call.
Testing Your Signature
Using the Bringg Signature Tester, you Can Test Your Public Key (access_token) and Private Key (secret_key).
If You Do Not Use Bringg API Calls You Do Not Need A Signature
Bringg Services, Bringg Webhooks, and the Bringg SDKs do not require a signature, because they do not require authentication.
Updated over 4 years ago